Compiling and Debugging
This chapter describes how to compile and debug multithreaded programs.
Compiling a Multithreaded Application
Many options are available for header files, define flags, and linking.
Preparing for Compilation
The following items are required to compile and link a multithreaded program. Except for the C compiler, all should come with your Solaris operating environment.
A standard C compiler
Include files:
The Solaris threads library (libthread), the POSIX threads library (libpthread), and possibly the POSIX realtime library (librt) for semaphores
MT-safe libraries (libc, libm, libw, libintl, libnsl, libsocket, libmalloc, libmapmalloc, and so on)
Choosing Solaris or POSIX Semantics
Certain functions, including the ones listed in Table 7-1, have different semantics in the POSIX 1003.1c standard than in the Solaris 2.4 Operating Environment release, which was based on an earlier POSIX draft. Function definitions are chosen at compile time. See the man Pages(3): Library Routines for a description of the differences in expected parameters and return values.
Table 7-1 Functions With POSIX/Solaris Semantic Differences
sigwait(2) |
|
ctime_r(3C) | asctime_r(3C) |
ftrylockfile(3S) - new | getlogin_r(3C) |
getgrnam_r(3C) | getgrgid_r(3C) |
getpwnam_r(3C) | getpwuid_r(3C) |
readdir_r(3C) | ttyname_r(3C) |
The Solaris fork(2) function duplicates all threads (fork-all behavior), while the POSIX fork(2) function duplicates only the calling thread (fork-one behavior), as does the Solaris fork1() function.
Including <thread.h> or <pthread.h>
The include file <thread.h>, used with the -lthread library, compiles code that is upward compatible with earlier releases of the Solaris Operating Environment. This library contains both interfaces--those with Solaris semantics and those with POSIX semantics. To call thr_setconcurrency(3THR) with POSIX threads, your program needs to include <thread.h>.
The include file <pthread.h>, used with the -lpthread library, compiles code that is conformant with the multithreading interfaces defined by the POSIX 1003.1c standard. For complete POSIX compliance, the define flag _POSIX_C_SOURCE should be set to a (long) value >= 199506:
cc [flags] file... -D_POSIX_C_SOURCE=N (where N 199506L) |
You can mix Solaris threads and POSIX threads in the same application, by including both <thread.h> and <pthread.h>, and linking with either the -lthread or -lpthread library.
In mixed use, Solaris semantics prevail when compiling with -D_REENTRANT and linking with -lthread, whereas POSIX semantics prevail when compiling with -D_POSIX_C_SOURCE and linking with -lpthread.
Defining _REENTRANT or _POSIX_C_SOURCE
For POSIX behavior, compile applications with the -D_POSIX_C_SOURCE flag set >= 199506L. For Solaris behavior, compile multithreaded programs with the -D_REENTRANT flag. This applies to every module of an application. .
For mixed applications (for example, Solaris threads with POSIX semantics), compile with the -D_REENTRANT and -D_POSIX_PTHREAD_SEMANTICS flags.
To compile a single-threaded application, define neither the -D_REENTRANT nor the -D_POSIX_C_SOURCE flag. When these flags are not present, all the old definitions for errno, stdio, and so on, remain in effect.
Note - Compile single-threaded applications, not linked with either of the thread libraries (libthread.so.1 or libpthread.so.1), without the -D_REENTRANT flag. This eliminates performance degradation incurred when macros, such as putc(3s), are converted into reentrant function calls.
To summarize, POSIX applications that define -D_POSIX_C_SOURCE get the POSIX 1003.1c semantics for the routines listed in Table 7-1. Applications that define only -D_REENTRANT get the Solaris semantics for these routines. Solaris applications that define -D_POSIX_PTHREAD_SEMANTICS get the POSIX semantics for these routines, but can still use the Solaris threads interface.
Applications that define both -D_POSIX_C_SOURCE and -D_REENTRANT get the POSIX semantics.
Linking With libthread or libpthread
For POSIX threads behavior, load the libpthread library. For Solaris threads behavior, load the libthread library. Some POSIX programmers might want to link with -lthread to preserve the Solaris distinction between fork() and fork1(). All that -lpthread really does is to make fork() behave the same way as the Solaris fork1() call.
To use libthread, specify -lthread before -lc on the ld command line, or last on the cc command line.
To use libpthread, specify -lpthread before -lc on the ld command line, or last on the cc command line.
Prior to Solaris 9, you should not link a nonthreaded program with -lthread or -lpthread. Doing so establishes multithreading mechanisms at link time that are initiated at runtime. These slow down a single-threaded application, waste system resources, and produce misleading results when you debug your code.
In Solaris 9 and subsequent releases, linking a nonthreaded program with -lthread or -lpthread makes no semantic difference to the program. No extra threads or LWPs are created and the main (and only) thread executes as a traditional single-threaded process. The only effect on the program is to make system library locks become real locks (as opposed to dummy function calls) and you pay the price of acquiring uncontended locks.
Figure 7-1 summarizes the compile options.
Figure 7-1 Compilation Flowchart
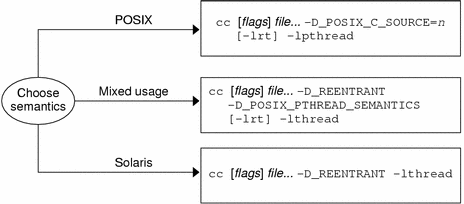
In mixed usage, you need to include both thread.h and pthread.h.
All calls to libthread and libpthread are no-ops if the application does not link -lthread or -lpthread. The runtime library libc has many predefined libthread and libpthread stubs that are null procedures. True procedures are interposed by libthread or libpthread when the application links both libc and the thread library.
Note - For C++ programs that use threads, use the -mt option, rather than -lthread, to compile and link your application. The -mt option links with libthread and ensures proper library linking order. Using -lthread might cause your program to core dump.
Linking With -lrt for POSIX Semaphores
The Solaris semaphore routines, sema_*(3THR), are contained in the libthread library. By contrast, you link with the -lrt library to get the standard sem_*(3R) POSIX 1003.1c semaphore routines described in "Semaphores".
Link Old With New
Table 7-2 shows that multithreaded object modules should be linked with old object modules only with great caution.
Table 7-2 Compiling With and Without the _REENTRANT Flag
The File Type | Compiled | Reference | And Return |
---|---|---|---|
Old object files (nonthreaded) and new object files | Without the _REENTRANT or _POSIX_C_SOURCE flag | The traditional errno | |
New object files | With the _REENTRANT or _POSIX_C_SOURCE flag | ||
Programs using TLI in libnsl1 | With the _REENTRANT or _POSIX_C_SOURCE flag (required) | The address of the thread's definition of t_errno. |
1 Include tiuser.h to get the TLI global error variable.
The Alternate libthread
The Solaris 8 Operating Environment introduced an alternate threads library implementation, located in the directories /usr/lib/lwp (32-bit) and /usr/lib/lwp/64 (64-bit). In the Solaris 9 Operating Environment, this implementation is the standard threads implementation found in /usr/lib and /usr/lib/64.
Debugging a Multithreaded Program
Common Oversights
The following list points out some of the more frequent oversights that can cause bugs in multithreaded programs.
Accessing global memory (shared changeable state) without the protection of a synchronization mechanism.